Sync
Allow multiple users to collaborate on the canvas with tldraw sync, an optional module included with tldraw.
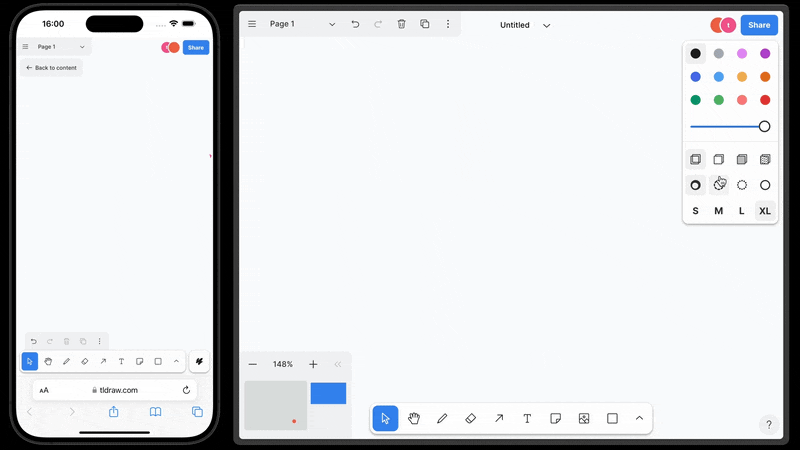
Changes to a document are synchronized in realtime to other users, including presence information like live cursors. This requires you to run a backend server, although we provide a demo server you can use for testing.
Try the demo server
The easiest way to start experimenting with multiplayer is with our demo server. Start by installing
@tldraw/sync
:
npm install @tldraw/sync
Then, in your app, call the useMutiplayerDemo
hook with a room ID. It'll return a
store
that you can pass into the tldraw component:
function MyApp() {
const store = useMultiplayerDemo({ roomId: 'myApp-abc123' })
return <Tldraw store={store} />
}
Make sure the room ID is unique. Anyone who uses the same ID can access your room. Our sync demo server is a great way to prototype multiplayer in tldraw, but it shouldn't be used in production. Rooms will be deleted after 24 hours.
Self-host the backend
To use tldraw sync in production, you need to host the backend on your own server. There are three parts to sync in tldraw:
- The sync server, which serves the room over WebSockets.
- Somewhere to large assets that are too big to send over websockets, like images and videos.
- The sync client, which connects to the server with the
useMultiplayerSync
hook.
We recommend starting from our Cloudflare Workers template. This is a minimal setup of the system that powers multiplayer collaboration for hundreds of thousands of rooms & users on tldraw.com. It includes everything you need to get up and running with multiplayer tldraw quickly: the sync server, asset hosting, and URL unfurling.
TLSocketRoom
The core part of our sync server is TLSocketRoom
. It works with any JavaScript-based web
framework with support for WebSockets, and any storage backend: it's up to you to wire in those
parts yourself.
Make sure there's only ever one TLSocketRoom
for each room in your app. If there's
more than one, users won't see each other and will overwrite others' changes. We use Durable
Objects to achieve this on tldraw.com.
If you don't want to our Cloudflare template, we also provide a simple Node/Bun server example. This is not production ready, but you can use it as a reference implementation to build it into your own existing backend.
Read the reference docs for TLSocketRoom
for more.
Asset storage
As well as synchronizing the rapidly-changing document data, tldraw also needs a way to store and retrieve large binary assets like images or videos.
TODO: implementing a server, implementing TLAssetStore, how much should live here vs in assets?
- Read about how assets work in tldraw.
- Read the
TLAssetStore
reference docs.
The sync client
- Bring it all together:
useMultiplayerSync
pointing to the new server and using theTLAssetStore
- User preferences & presence
- Default UX: offline indicator, people menu, cursors & cursor chat. point to overriding
Other sync systems
While tldraw multiplayer is the easiest way to add sync capabilities to your tldraw canvas, there are plenty of other sync systems that you may already be using. Here's some examples of how to integrate them.
Yjs
The CRDT library Yjs can be used as a sync engine for tldraw, as shown in the tldraw-yjs example.
TODO
- Partykit
- Liveblocks
- Automerge